space invaders
In this page I will showcase how I approached making space invaders!
Walls
I started off by making 1 wall then putting it on top of my camera view (which was doubled from 5 to 10). After that I doubled the wall to make the left side of my border and repeated the process for the right side and bottom side. I tried to make them be as equal as I can. I selected all walls in the hierarchy (shift + leftclick from top to bottom) and added "Box Collider 2D".
Following this, I ticked "is triggered" on every wall so that I can code this so my sprite will not go through the walls as it is moving.
Enemy
I went to the asset store and I looked for a space ship sprite and for an enemy sprite. I found some really great results which I ended up using. I made my enemy move through animator and it has a lot of animations going on for him. I did this by going into "Animator" (ctrl + 6) and selecting all cut up slices. The slices we're pre-cut as this is an asset from the Asset Store, so there was no need for me to go into Photoshop and create the enemy myself and then having to cut it.
I selected "Slime_0" until "Slime_15" with Shift+left-click from the first one to the last one. I then added this to "Animator" and changed the time as i see fit, with 1 second intervals.
Coding the enemy
I started off by creating a C# Script in the same folder as my sprite.
I want to script the enemy to move to the right meanwhile its animating and for it to then bounce back meanwhile remaining on the same axis. I added two script lines which are: "public float speed;" ; this will allow me to change the speed and it is public so i can access it from unity and "Vector2 direction;" this will make my sprite move in a certain direction.
After i entered these 2 script lines I needed to assign a direction to the variable I have entered before otherwise my "enemy" sprite would not move.
The script " direction = Vector2.right;" would inform the console that my sprite would move positively on the x axis. After this I went on to add another script line which would make my sprite move through transform and translate.
I added this script in the "void update" because this would be happening every frame/second of the game since it starts, then I saved this (Ctrl + s) . I then added a speed of 5 to my sprite which will now cause it to move.
I added the following attachments so my sprite does not go through the wall. I ticked the box "Is Trigger" and i changed gravity to 0. Ticking the box "Is Trigger" would stop my "enemy" from going through the wall and changing the gravity to 0 would mean that my "enemy" wouldn't go straight to the ground and not come up anymore, since it has no bounce effect.
I added some coding lines to trigger an event so that my "enemy" would go positively on the x axis and once it has hit a wall it would negatively go on the x axis. the line "OnTriggerEnter2D" is what will trigger the event and the following coding line "direction.x = -direction.x;" is what will actually tell it what to do once the event has been triggered.
Player
I have looked for a sprite for my ship as it would look way better than I could have made them in pixel art! I found a very interesting ship which i completely love and it looks very detailed. This is going to be my "player" but it will not be animated.
I went into "Project Settings" to set up the controls of my "player".
I changed the Axes size to 2, instead of 18 since I only want my player to move positively and negatively on the x axis. Also i want a button to make my player fire. I removed the negative buttons from the fire section because I would not need them. I want the player to only fire positively on the y axis.
Coding "player"
Since the "player" will move on the x axis i added a code line which will allow me to make it happen. "public float speed;" will allow me to add movement to my "player".
I added the first line of coding which indicates how my "player" will move and that it will even move when certain keys will be pressed; left arrow key and right arrow key. I then attached my script to my "player" and I changed my script's speed to 10 otherwise my "player" would not move at all.
I then added the line "public GameObject thebullet;" because I want the bullet to come shooting straight from the player and I want it to be cloned whenever I click space. I have also done a prefab for this which have copied all my "bullet" scripting,attachments and sprite animation
I have also added the code that when I click space it will shoot rather than it shooting every frame rate. I've done this by using the "if" statement. Also "Instantiate(thebullet, transform.position, transform.rotation); " code line basically means that the "bullet" will spawn where my player is at all times. Then I added my prefab to to my script so "thebullet" has a sprite and animation.
Bullet
My bullet's sprite was also taken from the asset store, the same place as I got the ship from as well. I wanted to have some animation to my bullet as it get shot. With my intervals on the animation it was a bit random because i wasn't too fussed about the bullet changing too fast. I wanted it to be quite slow so it wouldn't loop before it actually hit something because the bullet evolves in 3 different stages and once it hits stage 3 it would be weird for it to go back to stage 1 mid fight, it wouldn't really make sense. Then I attached this to my bullet.
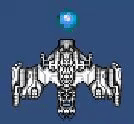
Bullet coding
Again i used the function of "public float speed;" and I also used "private Rigidbody2D rigidbody;". I used the following line "rigidbody = GetComponent<Rigidbody2D>();". I'm getting the rigidbody object (bullet) and storing it inside the Rigidbody (the player). Then I can access the properties of the bullet. The line "rigidbody.velocity = Vector2.up * speed;". This just gives the "bullet" some velocity to go upwards and multiplying it by speed.
I have also added this line of coding which will destroy the balls once the trigger event is triggered. (E.G hitting the wall.)
I gave all the walls a tag called "wall" and then I made a code that when the "bullet" collides with the wall it will trigger an event. This would completely destroy the bullets.
I also added a tag to the walls and a tag to the bullet. When the bullet would hit the "enemy" it would completely destroy it.
Background
I chose a very beautiful background for my game because even though it is called Space Invaders, if I really had time to work on this I would make it start off from earth flying away into space. I personally love these type of setting too because it reminds me of the morning. I love mornings and always have because it always reminds me of a new beginning.