Third Person Platformer
I start off by making a project Unity using the 3D template. i start off by making a plane to get ready to put my objects in place. I created a "Plane" object from the hierarchy.
Then I created a "Capsule" and it spawned in the middle of my "Plane". I then created a "Cylinder" and putting it on top of the "Capsule" and rotating it. Clicking the key R I started re-sizing it to make it smaller to fit my "Capsule". Following that I made the light a bit more dim to be able to actually see the player more clear and the "Plane". I then started to code on how to actually move my player which was done in Visual Studios.
I then went into "Project Settings" and I've changed the names of "Horizontal" to "Forwards" and "Vertical" to "Right" as to me it made more sense and came to me naturally more than the past names. I also removed the alternative buttons because otherwise the keys wouldn't have worked properly as i am using: W, A ,S and D. Some alternative keys were actually the same as the main keys, this would make it cancel each-other out = not working.
Before my coding I had to add an attachment to my player which is "Rigidbody".
I've entered:
- "Rigidbody rb;" - So that the console recognises "Rigidbody" as "rb"
- "public float speed;" - The variable that can be changed for the spped of the player. This is what also allows the player to move.
- "float forwardScale = Input.GetAxis("Forwards");" - This line of code is what allows my player to move when a certain key is clicked.
- "float rightScale = Input.GetAxis("Right");" - This line of code also makes my player move but a different direction and different keys are pressed to trigger this.
- "transform.position = transform.position + forwardScale * Vector3.forward + rightScale * Vector3.right;" - "transform.position = transform.position" is where the player is at the beggining before it would move and then the rest of the code is telling it how to move specifically.
I've entered:
- "Rigidbody rb;" - So that the console recognises "Rigidbody" as "rb"
- "public float speed;" - The variable that can be changed for the spped of the player. This is what also allows the player to move.
- "float forwardScale = Input.GetAxis("Forwards");" - This line of code is what allows my player to move when a certain key is clicked.
- "float rightScale = Input.GetAxis("Right");" - This line of code also makes my player move but a different direction and different keys are pressed to trigger this.
- "transform.position = transform.position + forwardScale * Vector3.forward + rightScale * Vector3.right;" - "transform.position = transform.position" is where the player is at the beggining before it would move and then the rest of the code is telling it how to move specifically.
I've also added " *speed Time.fixedDeltaTime;". I also changed the normal speed from 0 to 10 for my player to make it seem more of a natural movement.
Here is what I've gotten so far!
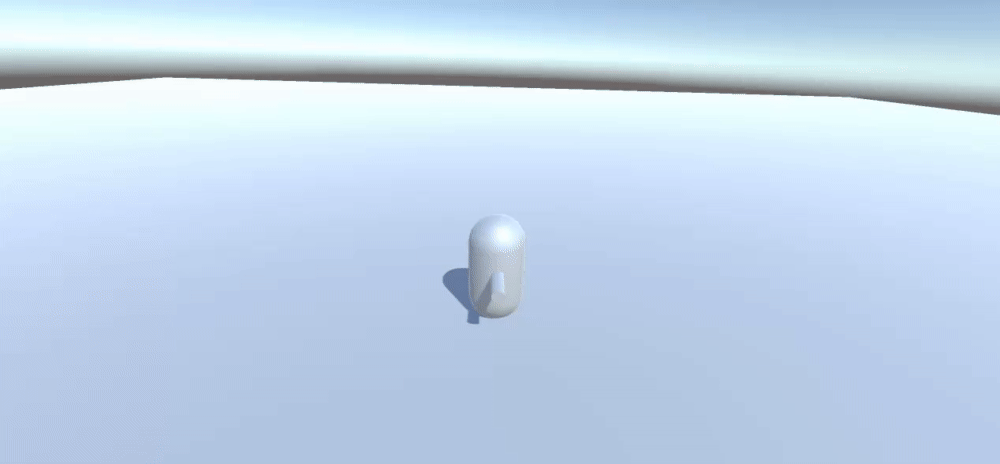
Rotation
Making it rotate only required 2 lines of coding which are:
- "public float turnSpeed;" - which would show "Turn Speed" in the UI of unity where the coding was placed. There I can change the speed of how fast it turns, I chose 10 because it felt the most natural.
- "transform.Rotate(0.0f , Input.GetAxis("Look") * turnSpeed, 0.0f);"
- "public float turnSpeed;" - which would show "Turn Speed" in the UI of unity where the coding was placed. There I can change the speed of how fast it turns, I chose 10 because it felt the most natural.
- "transform.Rotate(0.0f , Input.GetAxis("Look") * turnSpeed, 0.0f);"
I then changed some coding to make the character actually move the way that its facing.
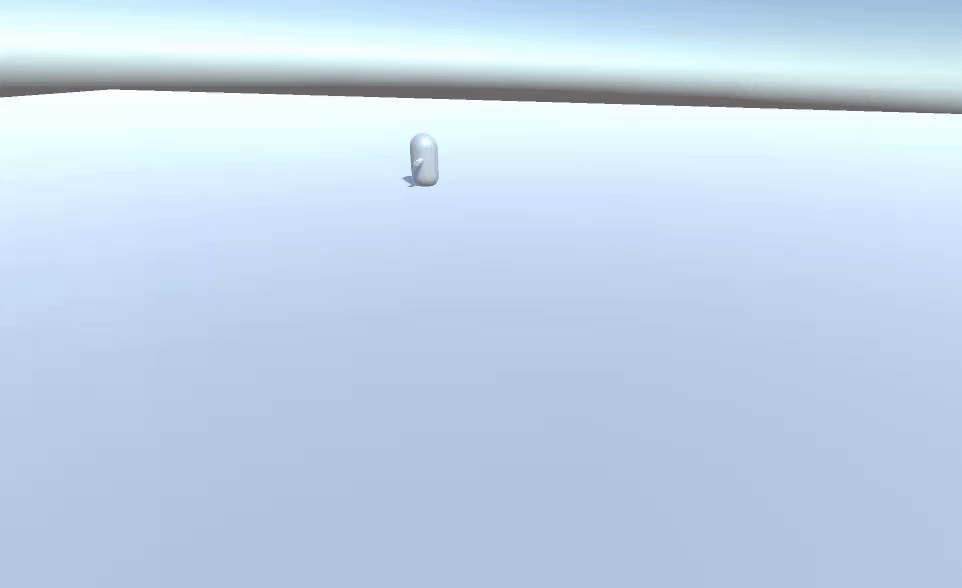
Jumping
I started off by going into "Project Settings" and changing "Fire 1" to "Jump" and attaching a key-bind to it which is "space". This is what will make the character jump.
I then started to add some variables to my coding so that the player has a jump force to jump with and to be able to enable it to jump with a trigger. This was done with "public float jumpforce;" and "bool jumpActive;".
Then I wrote this code for the jumping. This script means that when I click space the character will jump. Which is a trigger then the jumpActive would be true. I have also added a box to jump over as practice to test out my jump. I have also added a box collider to my character and I edited the box collider and put it at the bottom of my capsule.
Jumping results!
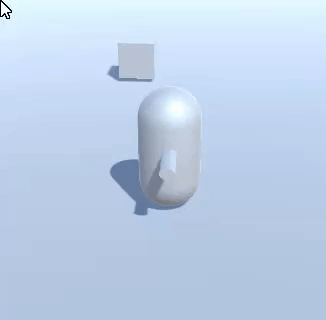
Camera follow
I created an "Empty" which then I named "CameraObjective". I made it a child of "CharacterPrototype" I will code it so that the "Main Camera" will follow the "CameraObjective" smoothly.
I coded this in such a way that the camera follows the "CameraObject". I dragged the "CameraObjective" and "CharacterPrototype" into their designated areas. I gave the Pos smooth time of 0.5 seconds to give it some depth and Ros Smooth Time 1 second for the same reason. If they would be the same it would be too overly repetitve.
With this code I wanted to achieve a more sophisticated way of the camera following the "CameraObjective" which would give it more detail rather than just a dead follow.
Final Result
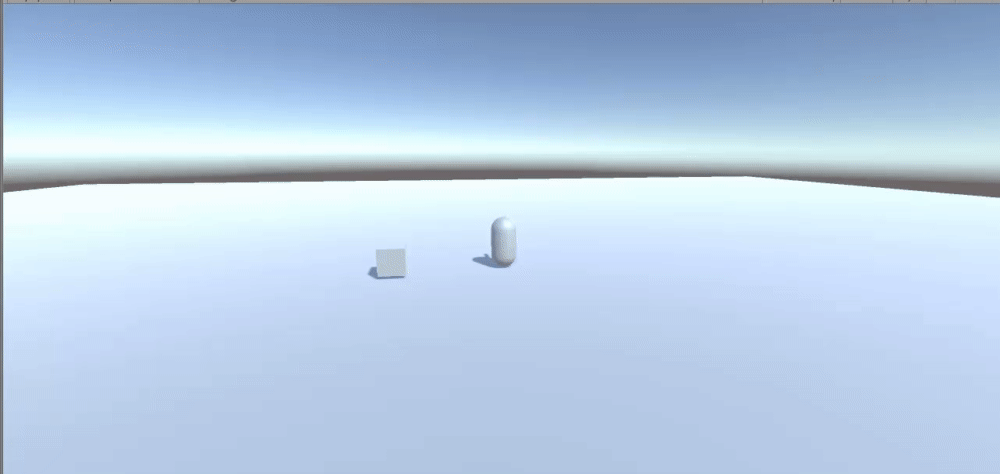